Level up your python skills : Essential tips and tricks for intermediate programmers
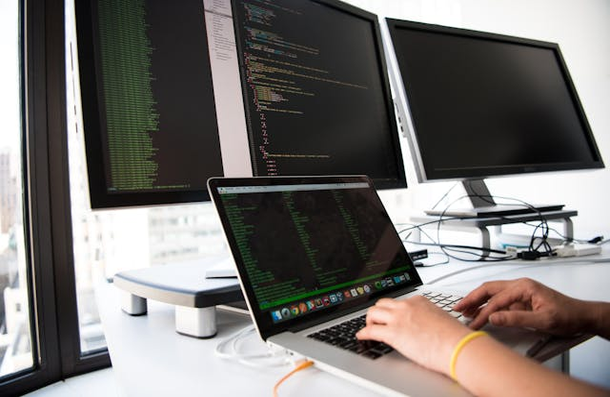
Python has become one of the most widely used programming languages not just because it’s readable or beginner-friendly, but because of the extensive ecosystem that surrounds it. From data science and automation to web development and machine learning, Python’s ecosystem is filled with mature, well-documented libraries that save time and extend the language’s core capabilities.
At the intermediate level, you’ll find yourself relying more on libraries beyond the standard set. Tools like itertools, collections, functools, and datetime offer much more than basic utilities. They’re often key to writing cleaner, faster, and more expressive code. Learning to incorporate them into your workflow can make even complex tasks feel more manageable and elegant. For web developers, Python offers powerful frameworks tailored to different project needs. Django is great when you want structure and speed—it gives you a lot out of the box and is ideal for building full-featured web applications. Flask, on the other hand, is lightweight and flexible, perfect for smaller apps or services that don’t need all the built-in features of Django. FastAPI is a more recent and exciting option, especially if you’re building APIs that require performance and scalability. It brings speed, strong typing, and modern development features into the picture.
When it comes to data handling, libraries like Pandas and NumPy become essential. They allow you to manipulate, analyze, and prepare data in a way that feels intuitive and efficient. Whether you're working with structured data, time series, or complex numerical computations, these libraries form the backbone of most data-related projects in Python. And for visualizing your findings, Matplotlib and Seaborn let you turn rows of data into graphs, charts, and visual stories that are easy to understand and share.
If your work leans toward machine learning or artificial intelligence, tools like Scikit-learn, TensorFlow, and PyTorch offer a wide range of capabilities for building predictive models, working with neural networks, and exploring complex algorithms. They’re designed to be flexible yet powerful, making it easier to build smart applications even without a deep background in AI.
The more familiar you become with these tools, the more efficient and confident your development process will feel. But it’s not about knowing everything. It’s about understanding where to look, what tools are available, and how to choose the right ones for each problem you face. That’s what sets intermediate developers apart—they don’t just write code, they build solutions with awareness and purpose.
Python’s Core Data Structures
Knowing how to use Python’s core data structures—lists, sets, dictionaries, and tuples—is essential, but as you advance, it becomes even more important to understand how to use them effectively. These structures are not just building blocks; they’re powerful tools that, when used well, can help you write faster, more efficient, and more readable code.
Lists are incredibly flexible. They’re great for ordered collections, and their dynamic nature makes them useful in a wide range of scenarios. But as you work with more data and write more complex code, you’ll want to think beyond just appending and iterating. Slicing, list comprehensions, and built-in methods like sort() and reverse() can help you work with lists in more efficient ways. You’ll also want to watch out for performance bottlenecks—lists can grow quickly, but certain operations (like inserting at the beginning) can be slower than expected.
Sets come in handy when you’re working with unique elements or need to perform operations like unions, intersections, and differences. They’re highly optimized for membership testing, making them a good choice when you're checking for duplicates or filtering large collections. The fact that sets are unordered and don’t allow duplicates can actually work in your favor when you need clean and distinct data.
Dictionaries are where things start to get really interesting. They’re fast, versatile, and ideal for mapping relationships between keys and values. As you move deeper into Python, dictionary comprehensions can help you transform and filter data in one clean line. Features like defaultdict and Counter from the collections module also open up new patterns that can simplify your code and reduce the need for extra conditionals or loops.
And while tuples may seem simple, they serve a valuable role in creating fixed collections or grouping related data together without the risk of accidental changes. They’re hashable, which makes them useful as keys in dictionaries or elements in sets—something lists can’t do. As your codebase grows, using immutable structures like tuples can help you prevent subtle bugs caused by unexpected changes to your data.
Writing Cleaner and More Optimized Python Code
Once you’re past the beginner stage, writing code that simply works is no longer the goal. Now it’s about writing code that works well—code that’s clean, efficient, and easy for both you and others to understand and build upon. That’s where optimization and clarity begin to matter just as much as functionality.
Clean Code:
Clean code is often underrated, but its impact is significant. It reduces bugs, improves collaboration, and saves time in the long run. Clear variable names, consistent formatting, and meaningful comments make a huge difference when returning to your code weeks or months later. Python has strong opinions on style, and the PEP 8 guidelines provide a solid foundation for writing code that’s not just correct but also readable.
Performance Optimization:
As for optimizing performance, Python offers several tools and techniques. List comprehensions are a good starting point—they’re more concise and often faster than traditional loops. Similarly, using built-in functions like map(), filter(), and zip() can simplify your logic and reduce overhead. Python is highly expressive, and leaning into that expressiveness often leads to more elegant solutions.
Profiling:
But writing fast code is not always about clever shortcuts. It’s about identifying real bottlenecks. That’s where profiling tools like cProfile, line_profiler, or timeit come in. They help you see where your program is spending time and what parts may need attention. Rather than optimizing everything blindly, profiling allows you to focus on the parts that actually impact performance.
Refactoring:
Refactoring is another skill that grows with experience. It involves restructuring your code to make it more efficient or readable without changing its behavior. Breaking functions into smaller, more focused pieces, removing duplicated logic, and avoiding unnecessary nesting are all simple refactoring steps that can improve your code’s clarity.
Efficiency:
Efficiency isn’t just about speed—it’s about maintainability. It’s about being intentional with every decision, avoiding unnecessary complexity, and writing code that does what it needs to without doing more than it should.
Mastering Object-Oriented Programming (OOP) in Python
Object-Oriented Programming (OOP) is essential for structuring larger Python projects. As an intermediate Python programmer, you’ll benefit from understanding the key concepts of OOP and how to apply them effectively in your code. OOP allows you to break down complex systems into manageable, reusable components.
Understanding the Basics of OOP in Python
Before diving into advanced OOP concepts, it’s important to solidify your understanding of the foundational principles. At its core, OOP revolves around four main principles: Encapsulation, Abstraction, Inheritance, and Polymorphism. These principles allow you to model real-world systems with objects that have attributes (properties) and methods (functions).
Classes and Objects:
A class is a blueprint for creating objects. An object is an instance of a class. In Python, classes are defined using the class keyword.
class Car:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
def display_info(self):
return f"{self.year} {self.make} {self.model}"
Here, Car is the class, and car1 is an object of that class.
Encapsulation:
This refers to bundling the data (attributes) and methods that operate on the data into a single unit, or class. It also involves restricting access to some of an object’s components to prevent accidental modification. This is done through private and public access modifiers (using underscore _ or __).
Abstraction:
Abstraction hides the complex implementation details and shows only the essential features of the object. You can achieve abstraction in Python through abstract classes or by simply designing classes with the interface in mind.
Advanced OOP Techniques in Python
Once you’ve got the basics of classes and objects down, you can begin exploring more advanced OOP techniques.
Inheritance
This is one of the most powerful aspects of OOP. Inheritance allows one class to inherit the attributes and methods from another, enabling code reuse and creating a hierarchical class structure. In Python, inheritance is simple to implement and allows for more flexible designs.
class ElectricCar(Car):
def __init__(self, make, model, year, battery_size):
super().__init__(make, model, year)
self.battery_size = battery_size
Here, ElectricCar inherits from Car, allowing it to access the display_info method while adding its own specialized feature (battery_size).
Polymorphism
This allows for using a single method name to perform different tasks depending on the object calling it. Method overriding is a typical example of polymorphism in Python.
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
In this example, both Dog and Cat override the speak method from the base Animal class.
Debugging Techniques Every Intermediate Programmer Should Know
At some point in your programming journey, you will encounter bugs in your code. Debugging is an essential skill for every developer, and as you transition into intermediate Python programming, having effective debugging techniques at your disposal becomes crucial. Python offers a rich set of tools to help you troubleshoot and identify issues in your code efficiently.
One of the most commonly used debugging tools in Python is the pdb (Python Debugger). This tool allows you to step through your code line by line, inspect variables, and evaluate expressions at runtime. By inserting the command import pdb; pdb.set_trace() into your code, you can pause execution and enter the interactive debugger. This enables you to check the state of your program at any given point, making it easier to spot issues like incorrect variable values or logical errors.
In addition to pdb, Python’s logging module is another invaluable tool for debugging, especially for more complex applications. Unlike print statements, which can clutter the output, logging allows you to output messages with different severity levels (e.g., DEBUG, INFO, WARNING, ERROR, CRITICAL). By setting appropriate logging levels, you can track the flow of your program and identify potential problems without overwhelming your output.
For quick and simple issues, print debugging can still be useful. This technique involves inserting print() statements at critical points in your code to track the values of variables and monitor the flow of execution. While not as sophisticated as pdb or logging, print debugging can be an effective approach when you’re just trying to understand what’s going wrong in a small section of code.
When working with more complex projects, it’s important to have an understanding of unit testing and test-driven development (TDD). While debugging is about fixing issues in your existing code, unit testing helps you ensure that your code behaves as expected in the first place. By writing test cases for individual functions or components, you can catch issues early in the development process, rather than after they’ve had a chance to compound into bigger problems. TDD takes this a step further by encouraging you to write tests before you write the actual code, promoting a mindset of building code with quality in mind from the start.
Another useful debugging technique is static code analysis, which involves using tools to analyze your code without executing it. Tools like pylint or flake8 help identify potential issues such as syntax errors, unused variables, or coding style violations, even before running the program. These tools can act as an early warning system, helping you catch errors before they become problematic.
Mastering these debugging techniques, will help you handle Python errors with confidence and efficiency, leading to smoother development cycles and more reliable applications.
Understanding Virtual Environments: Why You Need Them
One of the challenges that intermediate Python developers often face is managing project dependencies and ensuring that their environment remains consistent and isolated. As your projects grow, you'll find that different projects may require different versions of libraries or packages. This is where virtual environments come in. Virtual environments allow you to create isolated environments for each project, so that dependencies for one project do not interfere with those of another.
A virtual environment is a self-contained directory that contains a Python installation along with any packages that are installed in it. By using virtual environments, you can isolate your project’s dependencies from other projects or from the global Python environment. This ensures that each project has exactly the libraries it needs, without conflicts between versions.
For example, if one project requires version 1.2 of a package and another requires version 2.0, a virtual environment allows you to manage both versions separately, avoiding conflicts.
To create a virtual environment in Python, you can use the venv module, which is included in Python’s standard library starting from version 3.3. To create a virtual environment, run the following command:
python -m venv myenv
This will create a new directory called myenv that contains a fresh Python environment.
You can activate the environment by running:
# On Windows
myenv\Scripts\activate
# On macOS/Linux
source myenv/bin/activate
Once the environment is activated, you can install any packages needed for your project without affecting the global Python environment.
Comparing Virtualenv, Pipenv, and Conda
There are several tools available to help you manage virtual environments and dependencies. While virtualenv and pipenv are popular choices for Python projects, conda is also widely used, especially in data science and machine learning applications. Let's look at each tool and its features:
Virtualenv
virtualenv is a tool used to create isolated Python environments. It is lightweight and simple to use, making it a popular choice for many developers. The tool allows you to create multiple environments for different projects and manage dependencies independently for each one.
To install virtualenv, run:
pip install virtualenv
Then, create a new environment with:
virtualenv myenv
Activate the environment as discussed earlier, and you can start installing your project dependencies.
Pipenv
Pipenv is a more modern tool that combines the functionality of virtualenv with a package manager. It automatically creates a virtual environment for your project and manages your dependencies in a Pipfile and Pipfile.lock. This makes it easier to manage packages and ensure reproducibility across environments.
To install pipenv, run:
pip install pipenv
With pipenv, you can install dependencies using:
pipenv install
It also creates a Pipfile.lock, which ensures that everyone working on the project uses the same package versions, improving consistency and preventing compatibility issues.
Conda
Conda is a cross-platform package manager that can be used to create isolated environments and manage dependencies. While it is commonly associated with data science and machine learning due to its support for managing non-Python dependencies (like R or Julia), it’s also useful for general Python development.
To install conda, you typically install the Anaconda or Miniconda distribution. Once installed, you can create a new environment with:
conda create --name myenv python=3.8
To activate the environment:
conda activate myenv
Conda also makes it easy to install a wide range of packages, including those outside the Python ecosystem.
Best Practices for Managing Dependencies
When managing dependencies in your projects, it’s important to keep a few best practices in mind to ensure your environment is clean, reproducible, and easy to maintain:
- Use a requirements.txt file for Python packages: Even if you're using pipenv or conda, it’s a good idea to have a requirements.txt file in your project directory. This file contains a list of all the packages your project depends on, making it easier to set up the environment on a new machine. You can generate the file by running:
pip freeze > requirements.txt
To install the dependencies from requirements.txt, run:
pip install -r requirements.txt
- Pin your dependencies: Always specify exact versions of the packages you are using to avoid conflicts and issues down the line. For example, instead of just specifying requests, specify requests==2.24.0 to ensure that you’re always using the same version.
- Keep your environment updated: Regularly update your environment to benefit from security patches and performance improvements. With pipenv, you can use the pipenv update command to update your dependencies, and with conda, you can use conda update.
- Isolate your environments: Avoid installing packages globally unless absolutely necessary. Each Python project should have its own virtual environment to prevent version conflicts and keep your workspace clean.
The Importance of Testing Your Code
One of the most critical aspects of becoming a proficient Python programmer is understanding the importance of testing. While writing code is essential, ensuring that your code works correctly is equally important. As projects scale, manual testing becomes cumbersome, and bugs inevitably creep in. This is where automated testing comes into play. By writing tests for your code, you not only verify that it works as expected but also make it easier to maintain, debug, and refactor.
Python comes with a built-in testing framework called unittest. This module allows you to write simple test cases that automatically verify the functionality of your code. Testing early and often is one of the most reliable ways to ensure the quality and stability of your project. By writing unit tests, you’re able to isolate specific functionality, verify edge cases, and ensure that future changes to your codebase won’t break existing features.
Unit Testing with the unittest Framework
Unit testing is the foundation of any automated testing strategy. It involves writing tests that check the smallest units of your code, such as individual functions or methods, to ensure they produce the expected output. Python's unittest framework provides an easy way to create and run these tests.
To use unittest, you typically define a test class that inherits from unittest. TestCase. In this class, you’ll write methods that test different aspects of your code. Here’s a simple example:
import unittest
# The function we want to test
def add_numbers(a, b):
return a + b
# Our test case class
class TestAddNumbers(unittest.TestCase):
def test_add_two_numbers(self):
self.assertEqual(add_numbers(1, 2), 3)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -2), -3)
def test_add_zero(self):
self.assertEqual(add_numbers(0, 5), 5)
if __name__ == "__main__":
unittest.main()
In this example, we have a simple function, add_numbers, and a corresponding test case class TestAddNumbers. Each test method calls the function and uses assertions like assertEqual to check if the output matches the expected result. If all tests pass, you know your function behaves as expected.
Running this test suite will give you clear feedback about whether your code works as intended. You can run the tests from the command line using:
-m unittest test_file.py
This will execute all the test cases in the specified file and display the results.
Test-Driven Development (TDD)
Test-Driven Development (TDD) is a software development approach where you write tests before writing the actual code. The idea is simple: write a test that fails, then write the code that makes the test pass. This cycle—Red-Green-Refactor—helps ensure that your code is thoroughly tested from the start.
The process goes as follows:- Red: Write a test that fails because the functionality is not implemented yet.
- Green: Write just enough code to make the test pass.
- Refactor: Once the test is passing, clean up the code, ensuring it remains efficient and readable.
TDD encourages writing minimal, focused code that addresses specific requirements. It forces you to think about the design of your code before implementing it, which often leads to cleaner, more modular code.
Integration and Functional Testing
While unit tests are vital, they only cover individual components of your program. For more complex applications, you need to test how different parts of your system work together. This is where integration testing comes in.
Integration tests ensure that various modules or systems work together as expected. For example, you might test whether a database interaction works correctly with the user interface of your application. These tests typically focus on how different parts of your program interact and verify that the entire system works cohesively.
For example, when testing a web application, you might want to test how your Python code interacts with an external API or database. These tests are more extensive than unit tests and require careful setup of the environment to ensure everything is connected properly.
Another form of testing that complements unit and integration tests is functional testing. These tests verify that the software behaves according to the specified requirements from an end-user perspective. While unit tests check individual functions, functional tests ensure that the system works as a whole from a user's point of view.
Using Mocking and Test Fixtures
In more complex applications, you might need to isolate certain parts of your code during testing. For example, you might not want to call a live API during a test because it could introduce delays or dependencies that aren't relevant to your test case. In such situations, mocking is a powerful technique.
Mocking allows you to simulate the behavior of complex objects and external systems. Python’s unittest.mock module provides tools to replace parts of your code with mock objects during testing. This ensures that tests remain focused on the functionality you're testing, without depending on external systems.
Additionally, test fixtures are used to set up and tear down any resources or configurations needed for the tests. This could include creating mock databases, initializing external APIs, or preparing any necessary data.
Wrapping up
Mastering Python as an intermediate programmer is a journey of refinement, discovery, and consistent growth. It's about stepping beyond the basics and learning how to think more strategically with your code — understanding the tools available to you, using better structures, writing cleaner, more efficient scripts, and knowing how to troubleshoot problems with confidence. It’s in these small but powerful improvements that you build a deeper relationship with the language.
Key Takeaways
- Unit testing is an essential part of Python programming, helping to ensure individual components of your code work as expected. It’s best practice to use Python’s built-in unittest module to automate and organize your tests.
- Test-Driven Development (TDD) encourages writing tests before code, promoting a better design and ensuring your code works from the start.
- Integration testing is crucial for ensuring that different parts of your application work seamlessly together. It’s especially important for larger projects that involve complex interactions between modules.
- Continuous Integration (CI) automates the process of running tests every time new code is added. This ensures consistent code quality and reduces the chances of introducing bugs.
- Mocking is a technique that simulates external dependencies, allowing you to test code in isolation without interacting with real services or systems.
- Automating tests and setting up regular test runs in your CI pipeline ensures that your code is continuously validated, reducing manual effort and maintaining high-quality code.
- Test coverage tools help identify which parts of your code are covered by tests, ensuring no critical functionality is left untested.
Want to Build Something Amazing?
We prioritize your business success and we deliver faster. Our services are custom and build for scale.
Start nowMore Articles
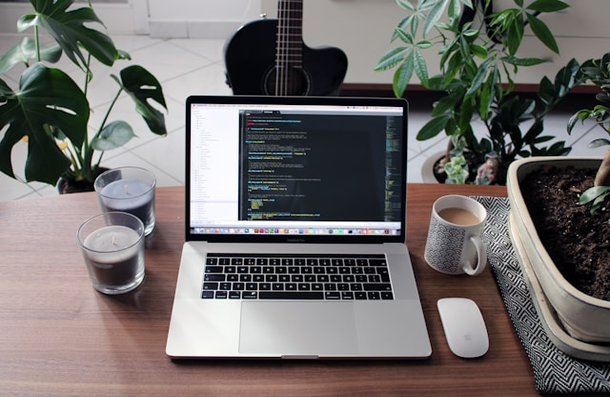
Introduction to HTML and CSS: Everything You Need to Know
3 weeks ago · 7 min read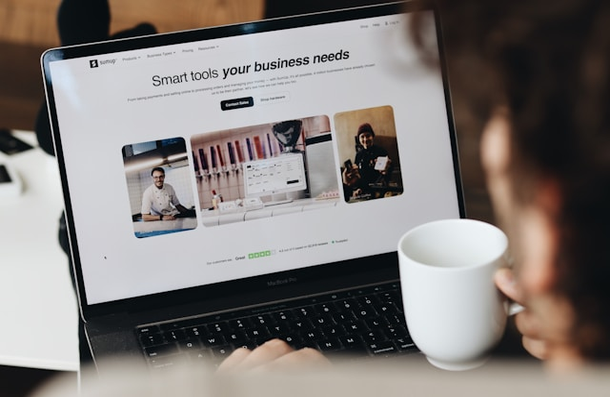
How to Promote Your Business Website in Nigeria
1 month ago · 5 min read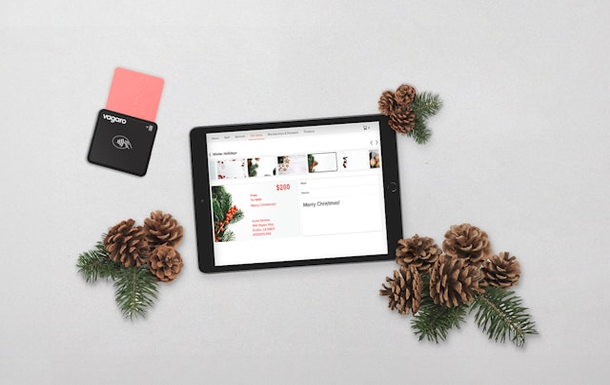
Boost your Online Sales with 16 Must-Have Features for Every Nigerian E-Commerce Website
1 month ago · 8 min read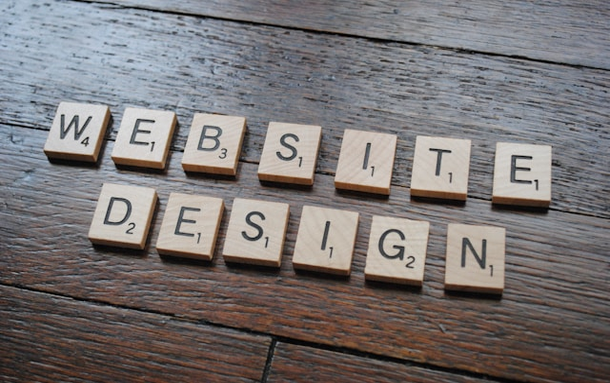
Hiring a Web Designer in Nigeria: Tips for Choosing the Best
1 month ago · 6 min read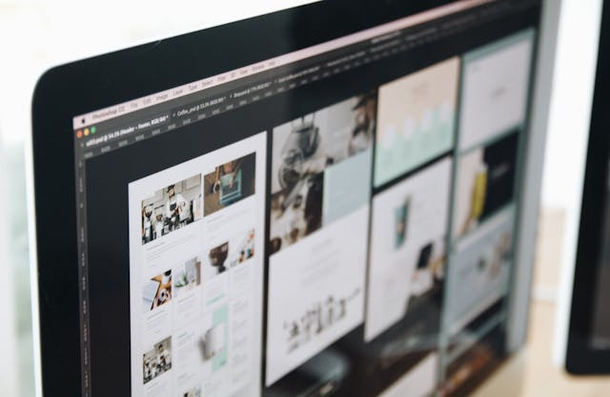
Nigerian business website redesign
1 month, 2 weeks ago · 4 min read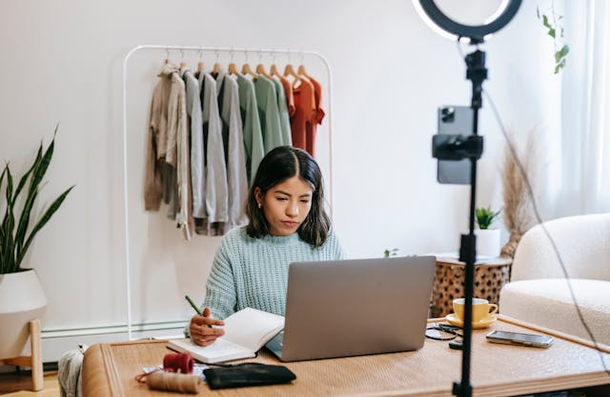
Benefits of blogging for Nigerian businesses
1 month, 2 weeks ago · 7 min read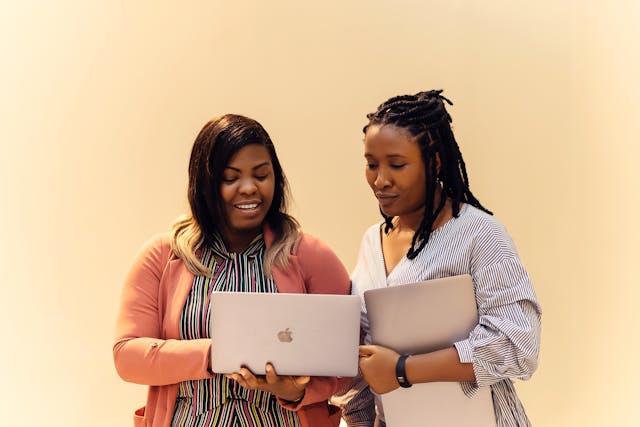
A guide to professionalism for Nigerian Small Businesses
1 month, 3 weeks ago · 6 min read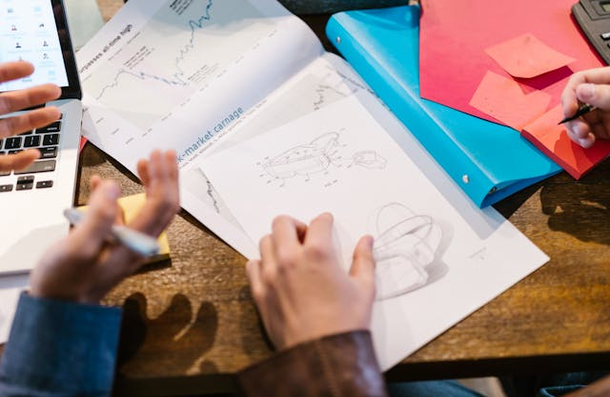
How to build a small business on budget
1 month, 3 weeks ago · 7 min read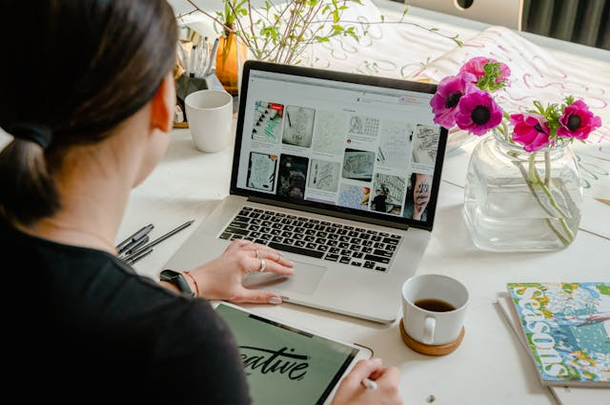
7 Website Mistakes Nigerian Business Owners must avoid for Online Success
1 month, 3 weeks ago · 7 min read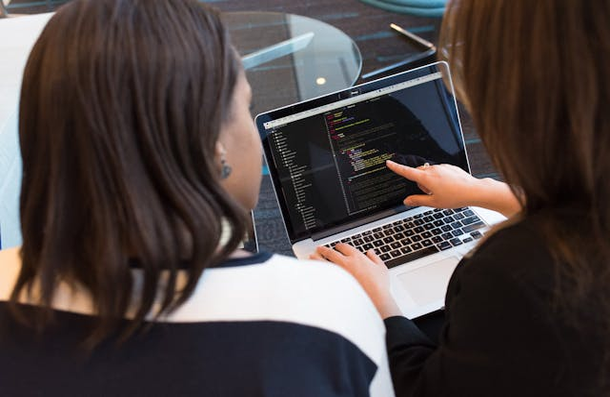
Mastering Algorithms and Data Structures for Coding Interviews
1 month, 4 weeks ago · 6 min read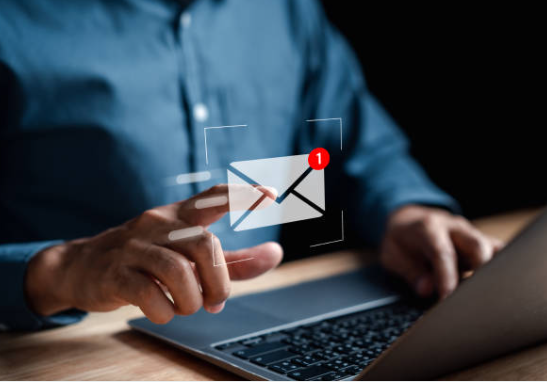
x